Python Tutorial - Static, Class and Regular Methods
This section of our beginning python training class always stumps students. Firstly, because they need to know the difference between a function and a method. Secondly, they need to understand object oriented programming concepts. Thirdly, they need to realize that python has three types of methods. Then they need to know how to use each method, which means they need to know the purpose of each method type. Then they have to understand mutable versus non-mutable types. The list goes on. As part of our python tutorial, I hope to shed some light on this confusing topic.
To begin, the difference between a function and a method in python is that a method is defined within a class. Here is an illustration:
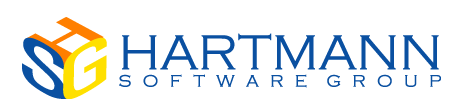
#function def greeting(): print "Hello, I hope you're having a great day!" class HSGPrinter(object): #method def greeting(self): print "Hello, I hope you're having a great day!"
As should be obvious, the second definition of greeting is encapsulated within the HSGPrinter class and is , therefore, refered to as a method.
The astute reader will notice that the greeting method contains one parameter named self. For those who know C++ , Java or C#, self is equivalent to this i.e. it is a reference to the invoking object:
hsgPrinter = HSGPrinter()
hsgPrinter.greeting()
Here hsgPrinter is the invoking object and, therefore, equivalent to self.
Now that we know the difference between a function and a method, let's focus on method types. The defined method is known as a regular or instance method because it contains a reference to self. Static and class methods do not contain this parameter. Moreover, they must be registered with the python runtime engine as such types. Here is the way it's done:
class HSGPrinter(object): count = 1 printertypes = ['HP','Canon'] #regular method def regularCounter(self): self.count = 4 print self.count self.printertypes.append('Lexmark') self.printertypes = ['Laser','Color'] print HSGPrinter.printertypes #class method def clsCounter(cls): cls.count = cls.count+1 print cls.count print HSGPrinter.printertypes clsCounter = classmethod(clsCounter) #static method def staticCounter(): print HSGPrinter.count print HSGPrinter.printertypes staticCounter = staticmethod(staticCounter) printer = HSGPrinter() printer.clsCounter() printer.regularCounter() printer.staticCounter()
Here is the outcome when running this module:
2
['HP', 'Canon']
4
['HP', 'Canon', 'Lexmark']
2
['HP', 'Canon', 'Lexmark']
The three methods from the example above are as follows:
1. regularCounter - regular method
a. has a self parameter
b. not registered with the python runtime engine in any special way
2. classCounter - class method
a. has a cls parameter which refers to the class itself. Used to access class variables
b. registered with the python runtime engine via the classmethod function
3. staticCounter - static method
a. contains no self or cls parameter
b. registered with the python runtime engine via the staticmethod function
When to use or create which method:
Regular methods are used to update and/or create object attributes or instance variables. This is accomplished using self as in self.count = 4. Remember that each object can have its own variables as opposed to class variables which are values common to all objects of a class. That is, a class variable is a variable that is referrenced by all objects such that the update of that variable is reflected to each object.
Class methods are used to handle class variables. They do not reguire the instantiation of an object to be invoked. Invocation of these methods is achieved by the class name preceding the class method name as in HSGPrinter.classCounter(). As a convenience, access to the class variables via these methods is accomplished by use of the cls or class parameter passed to this method as in cls.count.
Static methods have nothing to do with a class whatsoever. They are methods that are suited to replace functions which are seen in the object oriented programming world as dangling code. It is far more efficient and understandable to associate functionality with a class than with a function. Take for example a Math class, none of the methods should be regular methods because they require the instantiantion of an object. It is poor design to create a Math object in order to sum twon or more numbers. Moreover, there is hardly any reason to create class methods; other than say PI or E, how many other class variables are needed. The bulk of these methods should be static methods which may or may not need to access class variables and to whom are passed the requisite values to complete the computation. Such methods may be the cos, sign, arctan, addition, remainder ...
Here is the outcome of running the module above. Notice how the class variable is not modified by the expression self.count = 4. This variable only updates the objects count value and not the class' count value. Also, notice how the class variable printertypes is modified by the expression self.printertypes.append('Lexmark'). Why do you suppose this happened?
When modifying class variables that are mutable types, such as list, a new variable is not created as occured when changing a non-mutable number variable like count. Therefore, when writing self.count = 4, a new count variable is created for each object. On the other hand, when writing self.printertypes.append(...), the orginal class variable is modified instead of creating a printertypes variable for each object.
What happens in the following situation: self.printertypes = ['Laser','Color']? If you guessed that an object attribute or instance variable is created, you are correct. The class variable, printertypes, still exists, but each object also has its own such printertypes variable.
Access to each type is visible in the method shown here:
def printPrintertypes(self): print self.printertypes #object attribute print HSGPrinter.printertypes
Here is the outcome when running the method above:
['Laser', 'Color']
['HP', 'Canon', 'Lexmark']
other blog entries
Course Directory [training on all levels]
- .NET Classes
- Agile/Scrum Classes
- AI Classes
- Ajax Classes
- Android and iPhone Programming Classes
- Blaze Advisor Classes
- C Programming Classes
- C# Programming Classes
- C++ Programming Classes
- Cisco Classes
- Cloud Classes
- CompTIA Classes
- Crystal Reports Classes
- Design Patterns Classes
- DevOps Classes
- Foundations of Web Design & Web Authoring Classes
- Git, Jira, Wicket, Gradle, Tableau Classes
- IBM Classes
- Java Programming Classes
- JBoss Administration Classes
- JUnit, TDD, CPTC, Web Penetration Classes
- Linux Unix Classes
- Machine Learning Classes
- Microsoft Classes
- Microsoft Development Classes
- Microsoft SQL Server Classes
- Microsoft Team Foundation Server Classes
- Microsoft Windows Server Classes
- Oracle, MySQL, Cassandra, Hadoop Database Classes
- Perl Programming Classes
- Python Programming Classes
- Ruby Programming Classes
- Security Classes
- SharePoint Classes
- SOA Classes
- Tcl, Awk, Bash, Shell Classes
- UML Classes
- VMWare Classes
- Web Development Classes
- Web Services Classes
- Weblogic Administration Classes
- XML Classes
- Introduction to Spring 6, Spring Boot 3, and Spring REST
25 August, 2025 - 29 August, 2025 - Object Oriented Analysis and Design Using UML
20 October, 2025 - 24 October, 2025 - OpenShift Fundamentals
6 October, 2025 - 8 October, 2025 - ASP.NET Core MVC (VS2022)
7 July, 2025 - 8 July, 2025 - RHCSA EXAM PREP
16 June, 2025 - 20 June, 2025 - See our complete public course listing
did you know? HSG is one of the foremost training companies in the United States
Our courses focus on two areas: the most current and critical object-oriented and component based tools, technologies and languages; and the fundamentals of effective development methodology. Our programs are designed to deliver technology essentials while improving development staff productivity.
An experienced trainer and faculty member will identify the client's individual training requirements, then adapt and tailor the course appropriately. Our custom training solutions reduce time, risk and cost while keeping development teams motivated. The Hartmann Software Group's faculty consists of veteran software engineers, some of whom currently teach at several Colorado Universities. Our faculty's wealth of knowledge combined with their continued real world consulting experience enables us to produce more effective training programs to ensure our clients receive the highest quality and most relevant instruction available. Instruction is available at client locations or at various training facilities located in the metropolitan Denver area.
Upcoming Classes
- Introduction to Spring 6, Spring Boot 3, and Spring REST
25 August, 2025 - 29 August, 2025 - Object Oriented Analysis and Design Using UML
20 October, 2025 - 24 October, 2025 - OpenShift Fundamentals
6 October, 2025 - 8 October, 2025 - ASP.NET Core MVC (VS2022)
7 July, 2025 - 8 July, 2025 - RHCSA EXAM PREP
16 June, 2025 - 20 June, 2025 - See our complete public course listing
consulting services we do what we know ... write software
The coaching program integrates our course instruction with hands on software development practices. By employing XP (Extreme Programming) techniques, we teach students as follows:
Configure and integrate the needed development tools
MOntitor each students progress and offer feedback, perspective and alternatives when needed.
Establish an Action plan to yield a set of deliverables in order to guarantee productive learning.
Establish an Commit to a deliverable time line.
Hold each student accountable to a standard that is comparable to that of an engineer/project manager with at least one year's experience in the field.
These coaching cycles typically last 2-4 weeks in duration.
Business Rule isolation and integration for large scale systems using Blaze Advisor
Develop Java, .NET, Perl, Python, TCL and C++ related technologies for Web, Telephony, Transactional i.e. financial and a variety of other considerations.
Windows and Unix/Linux System Administration.
Application Server Administration, in particular, Weblogic, Oracle and JBoss.
Desperate application communication by way of Web Services (SOAP & Restful), RMI, EJBs, Sockets, HTTP, FTP and a number of other protocols.
Graphics Rich application development work i.e. fat clients and/or Web Clients to include graphic design
Performance improvement through code rewrites, code interpreter enhancements, inline and native code compilations and system alterations.
Mentoring of IT and Business Teams for quick and guaranteed expertise transfer.
Architect both small and large software development systems to include: Data Dictionaries, UML Diagrams, Software & Systems Selections and more